Learn How to Work with Strings in Python: Python is a popular programming language that is widely used in a variety of domains including data science, machine learning, web development, and more. One of the most important data types in Python is strings. In this article, we will cover how to work with strings in Python.
What is a String ?
A string is a sequence of characters enclosed in quotes, either single or double. For example, “Python Programming” and ‘String in Python’ are strings in Python. Strings are immutable, which means that once created, their contents cannot be changed.
How to declaring Strings in Python ?
You can declare a string in Python using either single or double quotes. For example:
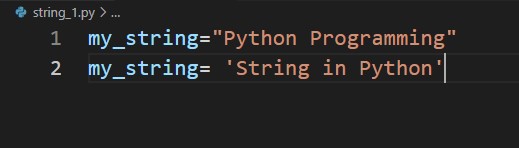
What is String concatenation ?
String concatenation in Python is the process of combining two or more strings into a single string. This is a common operation in programming when you need to build longer strings by combining shorter strings.
String concatenation is a useful technique in Python when you need to build longer strings from smaller ones, such as when creating messages, writing to files, or generating HTML or XML documents.
How to concatenate Strings in Python?
In Python, you can concatenate two or more strings using the “+” operator. For example:
This will output “Hello World”.
What is String Repetition in Python ?
In Python, string repetition refers to the process of repeating a string a certain number of times. This is achieved using the multiplication operator *.
To repeat a string a specified number of times, you can use the * operator and the number of repetitions.
In addition to repeating a string a fixed number of times, you can also use variables to specify the number of repetitions.
String repetition is a useful feature in Python that allows you to generate repeating patterns or perform certain operations a fixed number of times. It is often used in conjunction with string formatting to generate complex strings dynamically.
How to repeat a string in Python ?
You can repeat a string a certain number of times using the “*” operator. For example:
This will output “Hello Hello Hello”.
What is String Indexing in Python ?
String indexing refers to the process of accessing individual characters or substrings within a string by their position or index. The index of a string starts at 0 for the first character and increases by 1 for each subsequent character.
To access a character at a specific index in a string, you can use square brackets [] and the index of the desired character.
String indexing is a fundamental concept in Python that allows you to access and manipulate individual characters and substrings within a string. It is an important skill to master when working with strings in Python.
How to indexing string in Python ?
In Python, you can access individual characters of a string using indexing. The index starts at 0 for the first character and goes up to n-1 for the nth character, where n is the length of the string. For example:
Following will output:
H
W
What is String Slicing in Python ?
In Python, string slicing refers to the process of extracting a portion of a string by specifying the indices of the starting and ending positions. This is achieved by using the slicing operator [:] or substring() method.
How to Slicing String in Python ?
You can also extract a portion of a string using slicing. Slicing is done using the “:” operator, with the first number indicating the starting index and the second number indicating the ending index. The ending index is not inclusive, which means that the character at the ending index is not included in the slice. For example:
This will output:
“Hello”
“World”
What is String Methods in Python?
String methods are built-in functions that operate on strings and can be used to perform various operations such as manipulating, formatting, and searching strings. String methods are called on a string object using the dot notation syntax.
Here are some common string methods in Python are len(), upper(), lower(), strip(), split(), join(), replace(), startswith(), endswith(), find(), count() etc.
How to use String Methods in Python?
Python provides a number of built-in methods that can be used to manipulate strings. Here are some of the most commonly used string methods:
- len(): Returns the length of the string.
my_string = “Hello World”
print(len(my_string)) # Output: 11
- upper(): Converts all the characters in the string to uppercase.
my_string = “Hello World”
print(my_string.upper()) # Output: “HELLO WORLD”
- lower(): Converts all the characters in the string to lowercase.
my_string = “Hello World”
print(my_string.lower()) # Output: “hello world”
- strip(): Removes any leading or trailing whitespace characters from the string.
my_string = ” Hello World “
print(my_string.strip()) # Output: “Hello World”
- split(): Splits the string into a list of substrings, based on a specified delimiter. The default delimiter is whitespace.
my_string = “Hello World”
print(my_string.split()) # Output: [“Hello”, “World”]
- join(): Joins a list of substrings into a single string, using a specified delimiter.
my_list = [“Hello”, “World”]
delimiter = ” “
my_string = delimiter.join(my_list)
print(my_string) # Output: “Hello World”
What is String Formatting in Python?
In Python, string formatting refers to the process of creating a formatted string by substituting values or expressions into a placeholder within a string. This is a way to dynamically create strings that contain variable data.
How to use String Formatting in Python ?
Python provides several ways to format strings. Here are some:
- Using f-strings (formatted string literals): These are string literals that have expressions inside curly braces {}. The expressions inside the curly braces are evaluated and their values are inserted into the string. For example:
This will output “My name is Alice and I am 25 years old”.
- Using the format() method: This method takes one or more arguments and inserts them into the string at the specified positions. The positions are indicated by curly braces {}. For example:
This will output “My name is Alice and I am 25 years old”.
- Using %-formatting: This is an older way of formatting strings that is still supported in Python. It uses the % operator to format strings. For example:
This will output “My name is Alice and I am 25 years old”.
Conclusion
Working with strings in Python is essential, as strings are a fundamental data type used in many applications. In this article, we covered the basics of working with strings in Python, including declaring strings, concatenation, repetition, indexing, slicing, string methods, and string formatting. With these tools, you can manipulate and format strings in many different ways to suit your needs.
Read related Post:
- What is a list in Python Programming in Hindi ?
- What is tuple in Python Programming in Hindi ?
- What is Sequence in Python in Hindi ?
FAQs on String in Python
-
How do you convert a string to a list in Python ?
You can use the “split()” method to convert a string to a list in Python. By default, the “split()” method splits a string by whitespace characters, but you can also specify a separator character to use for splitting.
-
How do you check if a string contains a substring in Python ?
You can use the “in” keyword to check if a string contains a substring in Python.
-
How do you convert a string to an integer in Python ?
You can use the “int()” function to convert a string to an integer in Python.
-
How do you replace a substring in a string in Python ?
You can use the “replace()” method to replace a substring in a string in Python.
-
How do you remove whitespace characters from the beginning and end of a string in Python ?
You can use the “strip()” method to remove whitespace characters from the beginning and end of a string in Python.